**题目描述:**编写一个函数,检查输入的链表是否是回文的。
示例 1:
输入: 1->2
输出: false示例 2:
输入: 1->2->2->1
输出: true进阶:你能否用 O(n) 时间复杂度和 O(1) 空间复杂度解决此题?
1 | /** |
思路
本题同时考察了寻找链表中间节点、链表反转、判断两链表是否相同的知识点,把它们拆开来,就很好做了
- 采用快慢两个指针去寻找链表的中间节点;
- 根据链表的中间节点反转后一半的链表;
- 迭代比较链表前一半的元素和后一半的元素,判断节点的值是否相等,得出是否为回文
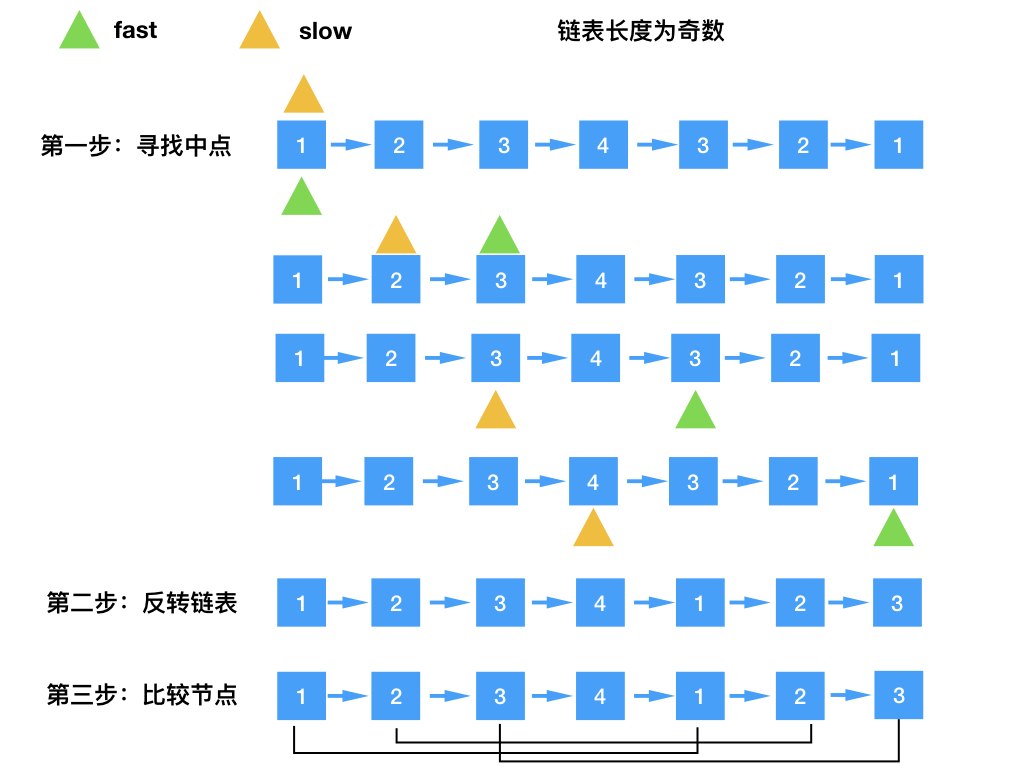
1 | class Solution { |
复杂度分析:
- 时间复杂度:O(n),其中 n 指的是链表的大小。
- 空间复杂度:O(1),我们是一个接着一个的改变指针,我们在堆栈上的堆栈帧不超过 O(1)。
执行结果:通过
执行用时:1 ms, 在所有 Java 提交中击败了99.93%的用户
内存消耗:42.4 MB, 在所有 Java 提交中击败了55.30%的用户